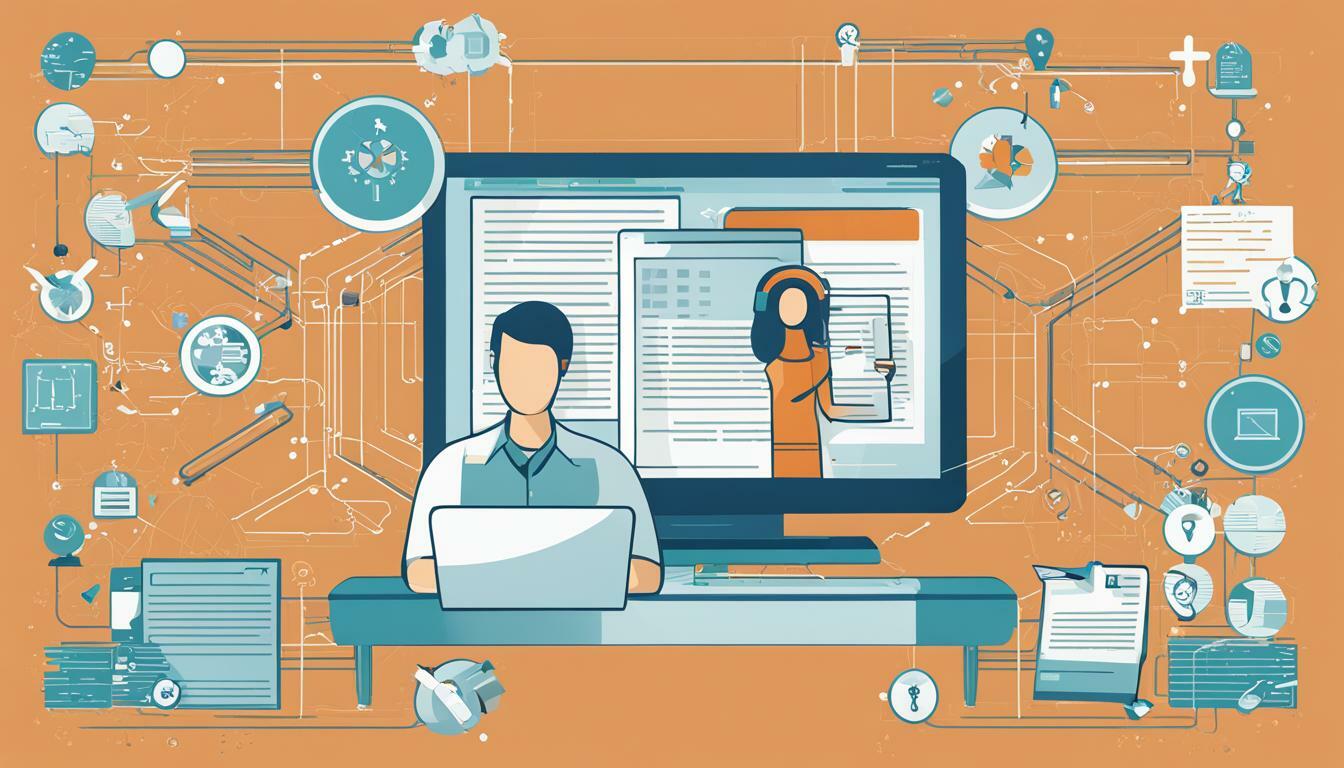
If you’re a developer looking to improve your API documentation, you’re in the right place. In this comprehensive guide, we’ll explore how to use Swagger and .NET Core to document APIs the Microsoft Way. By the end of this article, you’ll have the knowledge and skills to create clear and comprehensive API documentation that adheres to Microsoft’s standards.
Key Takeaways
- Swagger and .NET Core are powerful tools for documenting APIs.
- The Microsoft Way provides best practices and guidelines for API documentation.
- By following this guide, you’ll learn how to create high-quality API documentation using Swagger and .NET Core.
Understanding Swagger and .NET Core
If you’re working in the world of API documentation, it’s likely that you’ve heard of Swagger and .NET Core. These technologies are two of the most popular tools for creating clear and comprehensive API documentation. In this section, we will explore the basics of each technology and how they work together to optimize API documentation.
What is Swagger?
Swagger is a popular open-source framework that allows developers to document their APIs easily. With Swagger, developers can create interactive and user-friendly documentation that is easy to navigate, search, and understand. The framework provides a way to describe the structure and functionality of APIs so that developers and users can easily interact with them.
The Swagger documentation is based on the OpenAPI specification, which is a standard for defining RESTful APIs. With Swagger, developers can create documentation that is accurate, up-to-date, and consistent across all APIs.
What is .NET Core?
.NET Core is a popular open-source framework for building modern, cloud-based applications. This technology enables developers to create high-performance applications that can run on multiple platforms, including Windows, Linux, and macOS. .NET Core is an evolution of the .NET Framework and includes a set of reusable libraries, runtime, and compilers.
When it comes to API documentation, .NET Core is used to build the underlying API. The framework provides a way to create RESTful APIs that can be easily documented using Swagger.
How Do Swagger and .NET Core Work Together?
Swagger and .NET Core work seamlessly together to create comprehensive API documentation. .NET Core is used to create the API itself, and Swagger is used to document it. Within the .NET Core application, Swagger is added as a middleware. By default, Swagger reads the XML documentation file generated by .NET Core and creates documentation.
The Swagger documentation is also presented visually through a Swagger UI, which provides developers and users with an interactive way to interact with the API documentation. Using Swagger, developers can create an API documentation hub that is standardized, easy to use, and accessible to all users.
The Microsoft Way of Documenting APIs
Documenting APIs can be a cumbersome and time-consuming task, but it is an essential aspect of software development. Following Microsoft’s API documentation guidelines, commonly known as “The Microsoft Way,” can help ensure that your documentation is comprehensive, readable, and effective.
One of the tools that Microsoft recommends for documenting APIs is Swagger UI. This tool allows you to create interactive API documentation that is easy to navigate, understand, and test. Swagger UI works by parsing your API’s OpenAPI Specification (formerly called Swagger Specification), a machine-readable JSON or YAML file that defines your API’s endpoints, parameters, headers, request and response body, and errors.
Another tool that Microsoft endorses for documenting APIs is SwaggerHub, a cloud-based platform that provides collaborative design, development, and publishing of APIs. SwaggerHub allows you to store and manage your API’s OpenAPI Specification, invite team members to collaborate on the documentation, and generate various formats of documentation (HTML, PDF, Markdown, Swagger UI, etc.). SwaggerHub also integrates with popular tools like Git, GitHub, Bitbucket, and Jenkins, making the API documentation workflow seamless and efficient.
If you prefer to create or edit your OpenAPI Specification locally on your machine rather than in SwaggerHub, you can use Swagger Editor, a web-based editor that provides syntax highlighting, autocompletion, validation, and visualization of your OpenAPI Specification. Swagger Editor also allows you to import and export your specification in different formats, including JSON, YAML, and Swagger 2.0.
Microsoft Way API Documentation Guidelines | Swagger UI Features | SwaggerHub Benefits | Swagger Editor Capabilities |
---|---|---|---|
Clear and concise documentation | Interactivity | Collaboration and versioning | Syntax highlighting and validation |
Consistency and completeness | Navigation and searching | Integration with other tools | Autocompletion and visualization |
Accurate and up-to-date information | Testing and debugging | Multiple documentation formats | Import and export functionality |
By following the Microsoft Way of documenting APIs and leveraging the power of Swagger UI, SwaggerHub, and Swagger Editor, you can streamline your API documentation process and provide high-quality documentation for your API users.
Setting Up Swagger and .NET Core
Before documenting your APIs with Swagger and .NET Core, you need to set up the environment. Here are the step-by-step instructions for getting started:
- Install the Swashbuckle.AspNetCore NuGet package in your .NET Core web application.
- Configure Swagger in the ConfigureServices method of the Startup.cs file.
- Configure Swagger in the Configure method of the Startup.cs file.
- Run the application and navigate to the Swagger endpoint (/swagger) to see the generated Swagger UI.
It’s that simple to get started with Swagger and .NET Core for API documentation. Once you have everything set up, you can start customizing the documentation to meet your needs.
But wait, there’s more! With .NET Core, you have the option to generate OpenAPI Specification (formerly known as Swagger Specification) JSON files. These files can be used with other tools and services that support OpenAPI Specification for generating client code, testing APIs, and more.
To generate an OpenAPI Specification JSON file, add the UseSwagger and UseSwaggerUI methods to your Startup.cs file. Then, navigate to the JSON endpoint (/swagger/v1/swagger.json) to see the generated JSON file.
Now that you have Swagger and .NET Core set up, you’re ready to start documenting your APIs with ease and efficiency.
Documenting APIs with Swagger and .NET Core
Swagger and .NET Core provide developers with powerful tools to create comprehensive API documentation. When documenting APIs, it’s crucial to provide clear and concise information for other developers to use. Fortunately, Swagger and .NET Core make this process straightforward and efficient. Let’s explore how to document APIs step-by-step using Swagger and .NET Core.
Step 1: Define API Endpoints
The first step in documenting APIs with Swagger and .NET Core is to define the API endpoints. This involves identifying the HTTP methods and request and response payloads for each endpoint. You can use Swagger annotations to document the endpoints directly in your code. This makes it easy to keep your documentation up-to-date as you make changes to your API.
Step 2: Generate Swagger Documentation
After defining your API endpoints, the next step is to generate Swagger documentation. This can be done by adding the Swashbuckle NuGet package to your .NET Core project. Swashbuckle will automatically generate Swagger documentation based on the annotations you added in the previous step.
Step 3: Customize Swagger Documentation
Once you’ve generated Swagger documentation, you can customize it to fit your specific needs. Swagger provides a wide range of customization options, including changing the UI appearance, adding external documentation links, and specifying response codes.
Step 4: Host Your API Documentation
Finally, it’s time to host your API documentation. There are several options for hosting Swagger documentation, including using Swagger UI, SwaggerHub, or a custom documentation portal. Whatever option you choose, make sure to provide clear and easy-to-find links to your API documentation from your API endpoints.
Documenting APIs with Swagger and .NET Core doesn’t have to be a daunting task. By following these steps, you can create clear and comprehensive API documentation that other developers will find easy to use and understand.
Optimizing Swagger and .NET Core Documentation
Once you have created your API documentation using Swagger and .NET Core, it’s time to optimize it for maximum impact. The following techniques and strategies will help you create documentation that is easy to read, navigate, and understand:
1. Structure your documentation effectively
Organize your documentation into logical sections, with a clear table of contents that provides easy access to specific parts of your API. Use headings, subheadings, and bullet points to break up large chunks of text and make the information more digestible.
2. Improve readability
Use simple, easy-to-understand language, and avoid technical jargon whenever possible. Use visual aids, such as diagrams and charts, to help illustrate complex concepts. Be sure to format code snippets in a way that is easy to read and follow.
3. Ensure consistency throughout your documentation
Use a consistent style and format throughout your documentation, including headers, bullets, and text formatting. This will make it easier for users to navigate and understand your API documentation.
4. Include examples and use cases
Provide real-world examples and use cases that demonstrate how your API works in action. This will help users understand how to use your API and give them a concrete idea of how it can be used in their own applications.
5. Keep your documentation up-to-date
Make sure you keep your documentation up-to-date and accurate with any changes or updates made to your API. This will ensure that users always have access to the most current information and will help avoid confusion or errors.
Conclusion
Congratulations on completing the comprehensive guide to documenting APIs using Swagger and .NET Core, following the Microsoft Way. By now, you should have gained a solid understanding of how to optimize API documentation efficiency and enhance your coding skills.
Utilizing Swagger and .NET Core, you can document your APIs in a clear and comprehensive way, adhering to Microsoft’s standards. You can also configure Swagger to generate accurate and up-to-date documentation, improving the overall quality of your API documentation project.
Remember to structure your documentation effectively, improve readability, and ensure consistency throughout your API documentation project. By following the best practices and guidelines outlined in this guide, you can become proficient in documenting APIs the Microsoft Way.
Thank you for joining us on this journey to explore the power of Swagger and .NET Core in API documentation. We hope this guide has been valuable to you in your coding endeavors.
FAQ
Q: What is Swagger?
A: Swagger is an open-source software framework that allows developers to design, build, and document RESTful APIs. It provides a set of tools and specifications for creating interactive API documentation.
Q: What is .NET Core?
A: .NET Core is a cross-platform, open-source framework developed by Microsoft for building modern, cloud-based applications. It allows developers to create APIs that can run on various platforms, including Windows, Linux, and macOS.
Q: How does Swagger complement .NET Core for API documentation?
A: Swagger integrates seamlessly with .NET Core, providing a user-friendly interface for documenting APIs. It automates the process of generating API documentation and makes it easy to keep the documentation in sync with the actual API implementation.
Q: What are the benefits of using Swagger and .NET Core for API documentation?
A: Using Swagger and .NET Core for API documentation offers several benefits. It improves the efficiency of documenting APIs by automating the process and ensuring accuracy. It also enhances the developer experience by providing a user-friendly interface and promotes consistency in API documentation across different projects.
Q: Can I use Swagger with other frameworks besides .NET Core?
A: Yes, Swagger is a framework-agnostic tool and can be used with various programming languages and frameworks. It is widely supported within the API development community and can be integrated with different backend technologies.
Q: Are there any additional tools or resources that can be used alongside Swagger and .NET Core?
A: Yes, there are additional tools and resources that can enhance your Swagger and .NET Core API documentation process. Some popular options include Swagger UI, SwaggerHub, and Swagger Editor, which provide advanced features for designing, documenting, and managing APIs.